Introduction¶
What is Angular?¶
- A framework for building client applications in HTML, CSS and Javascript/ TypeScript
Benefits of Using Angular¶
- Gives our applications a clean structure
- Includes a lot of re-usable code
- Makes our applications more testable
- Angular makes your life easier!
Architecture of Angular Apps¶
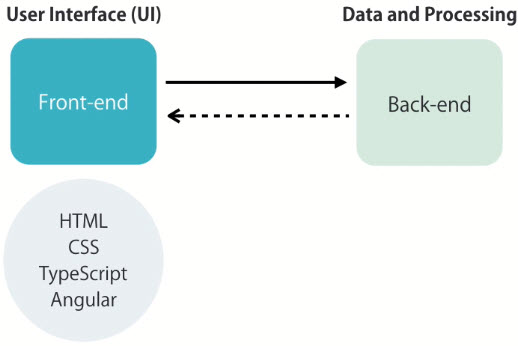
HTTP Services / APIs¶
- API stands for Application Programming Interface
- Endpoints that are accessible via the HTTP protocol
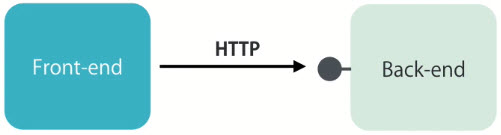
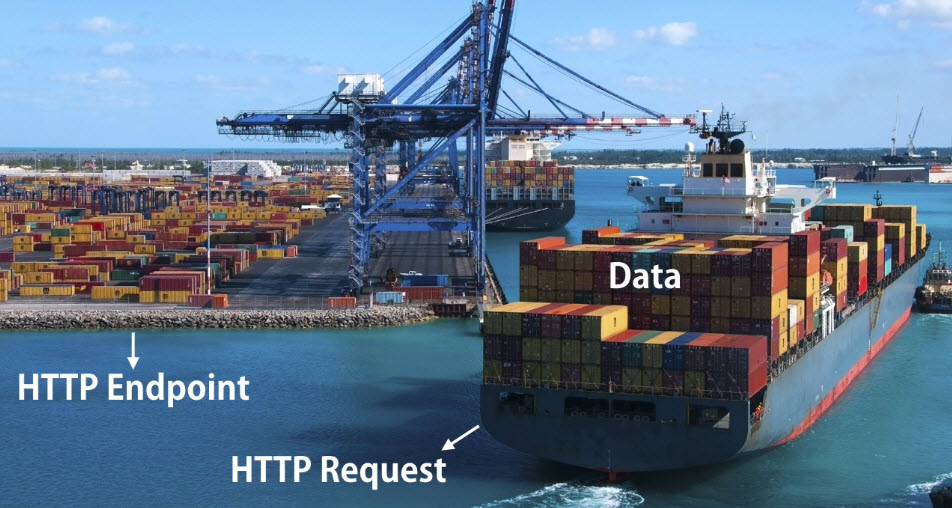
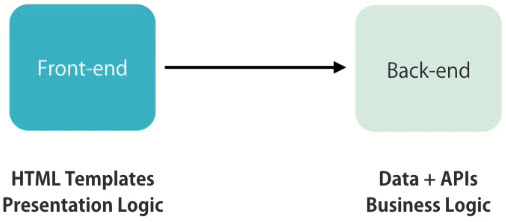
Setup the Development Environment¶
- Download and Install node.js
- https://nodejs.org/en/
- node.js is basically a runtime environment for executing Javascript code outside the browser
- Only use the Node Package Manager (NPM) in node.js to download libraries
- Install Angular CLI
- (admin)
npm install -g @angular/cli
- check version:
ng --version
- Create a New Project
- cd to your project folder
- (admin)
ng new hello-world
- Download and Install Visual Studio Code
- Open the Web Server
- At terminal, type
ng serve --open
- This will open up the server and listen to port 4200
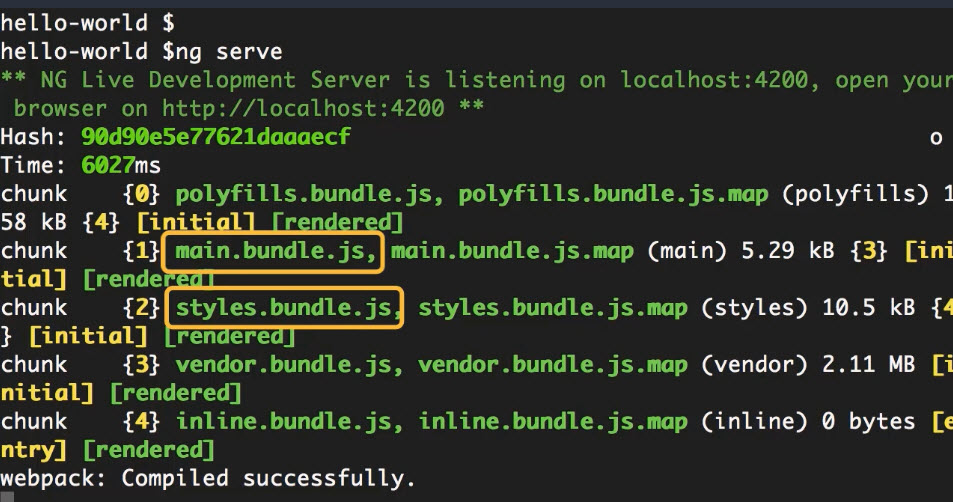
Structure of an Angular Project¶
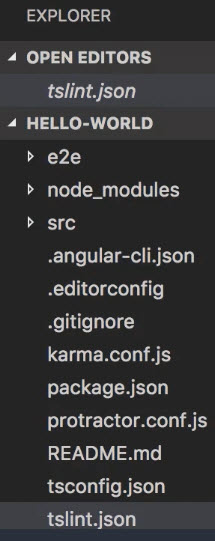
- e2e folder
- Stands for End-to-End
- Where we write end-to-end tests for our application
- These are basically automated tests that simulate a real user
- For example, we can write code to launch our browser navigate to the page, click a few links and fill out a form, click a button and then assert that there is something on the page
- node_modules folder
- Where we store all the third party libraries that our app may depend upon
- When we compile our app, part of this third party libraries are put in a bundle and deploy with our app
- src folder
- Where we have our actual source code of our app
- src > app folder
- Contains a module and a component files
- Every app has at least one module and one component
- src > assets folder
- Where we store the static assets of our app
- For example image and icon files
- src > environment folder
- Where we store configuration settings for different environments
- For example,
environment.prod.ts
for production environment and environment.ts
for development environment
- src > index.html
- Contains our app
- Note that we don't have any reference to a script or a stylesheet
- These references will be dynamically inserted into this page
- src > main.ts
- A TypeScript (ts) file which is basically a starting point of our app
- Just like a main method in our programming languages
- Bootstraping the main module of our app
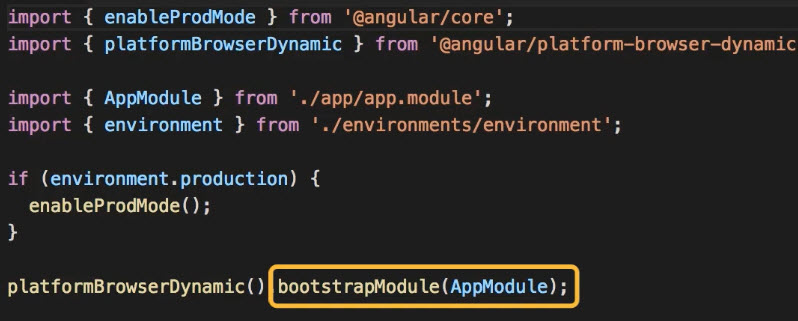
- Angular loads
AppModule
and everything else starts from there
- src > polyfills.ts
- Imports some scripts that are required for running Angular
- Because the Angular framework uses features of Javascript that are not available in the current version of Javascript supported by most browser
- These polyfills fill the gap between the feature of Javascript that Angular needs and the features supported by the current browsers
- src > style.css
- Contains the global styles of our app
- src > test.ts
- Used for setting up our testing environments
- .angular-cli.json
- Configuration file for Angular-CLI
- .editorconfig
- For working a team environment, you want to make sure that all developers in the team use the same setttings in their editors. This is where you store your settings
- .gitignore
- For excluding certain files and folders from your git repository
- Git is basically a tool for managing and versioning your source code
- karma.conf.js
- Configuration file for Karma which is a test runner of Javascript code
- package.json
- A standard file that every node project has
- Contains the basic settings of your app
- The key
dependencies
contains a list of dependencies i.e. the libraries that are required by your app
- The key
devDependencies
contains the libraries that we need in order to develop this app. These dependencies are not required to run on our production server
- protractor.conf.js
- A tools for running and to test for Angular
- tsconfig.json
- Contains settings for TypeScript compiler
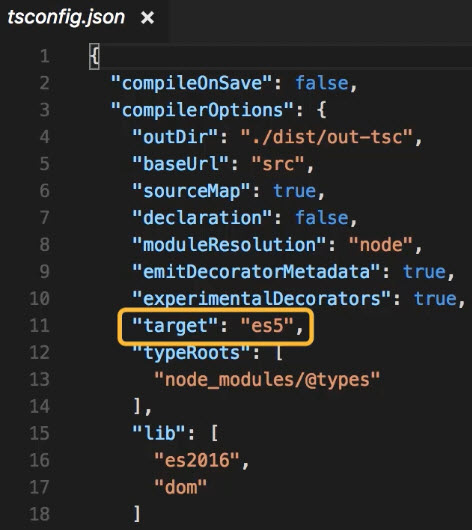
- tslint.json
- Contains settings for TsLint which is a static analysis tool for TypeScript code
- It checks your TypeScript code for readability, maintainability and functionality errors
WebPack¶
- Angular CLI uses a tool called webpack which is a build automation tool
- Whenever you change one of your stylesheet, TypeScript or HTML files, webpack automatically recompile your app and refresh your bundles. This feature is called Hot Module Replacement/ Reloading (HMR)

- Webpack gets all our scripts and stylesheets, combines them into a bundle and then minimize the bundle for optimization
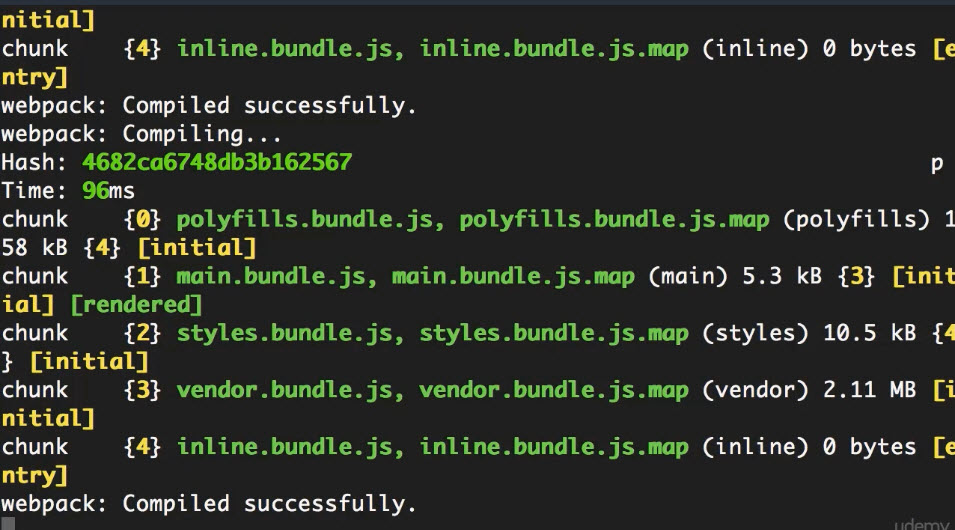
- For example vendor bundle "vendor.bundle.js" includes all third party libraries
- If you view page source, we will notice the following:

- All the bundles that webpack generated is also injected into index.html
- That's why we don't have any references on stylesheet or script tags in index.html
Course Structure¶
- Items in red are optionals
- You don't need the advanced topics for the Final Project
- For a shortcut, you can focus on the absolute essentials and then move to the final project
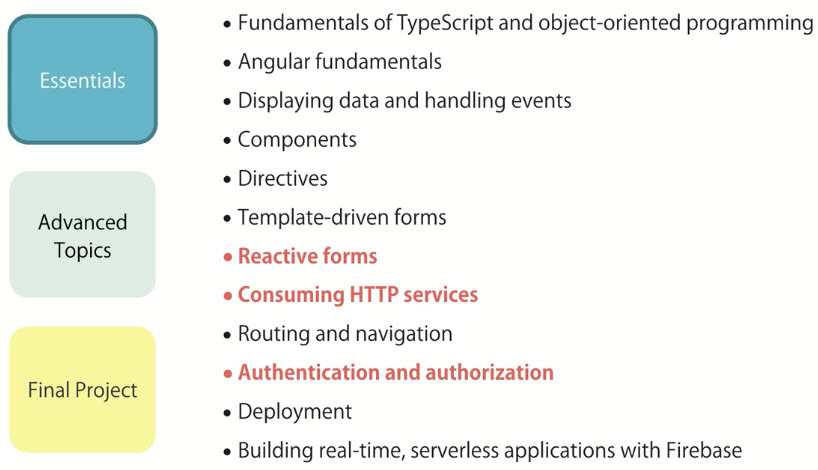
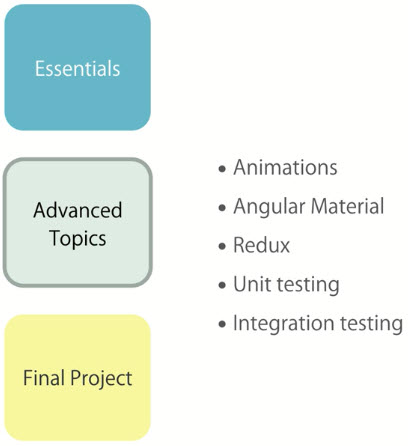